How to Use MySQL LEAD
and LAG Analytical Functions
LEAD and LAG functions are powerful analytical tools that are frequently used in MySQL, a popular database management system for many web applications. These functions are good for analyzing time series data, detecting patterns, and improving querying capabilities. By utilizing MySQL window functions, developers and database users can extract valuable insights from large datasets.
Understanding Window Functions in MySQL
In MySQL, window functions are used to perform calculations across a set of rows that are related to the current row. This can be incredibly useful when working with large datasets and can help simplify complex queries. The syntax for window functions in MySQL is as follows:
function_name() OVER ( [PARTITION BY partition_expression, ... ] [ORDER BY order_expression [ASC|DESC], ... ] [ROWS frame_specification] )
function_name
- can be any aggregate function such as SUM, AVG, COUNT, MIN, or MAX.partition_expression
- divides the rows into partitions.order_expression
- sorts the rows within each partition using the ORDER BY clause.frame_specification
- defines the range of rows over which the calculation is performed.-
LEAD
- can be applied to the rows within each partition to retrieve the value from the specified offset. It can be specified using either ROWS BETWEEN or RANGE BETWEEN, followed by UNBOUNDED PRECEDING, CURRENT ROW, or UNBOUNDED FOLLOWING.
Understanding window functions in MySQL is important for anyone working with large datasets and complex queries. You can simplify your queries and perform calculations across sets of related rows with ease. Now, let us take a closer look at LEAD and LAG analytical functions.
MySQL LEAD Function
The MySQL LEAD function is a powerful tool that can help you easily access data from the next row in a dataset. It is particularly helpful when working with time-series data, as it allows you to access values from the future without having to write complex SQL queries.
The syntax for the LEAD function in MySQL is as follows:
LEAD(expression, offset, default) OVER ( [PARTITION BY partition_expression, ... ] [ORDER BY order_expression [ASC|DESC], ... ] )
expression
- is a parameter that specifies the column or expression to retrieve the value for.offset
- specifies the number of rows to look ahead.default
- is an optional value that is returned if there are no rows available for the specified offset.PARTITION BY
andORDER BY
clauses are used to define the partitions and sort order for the rows, respectively.LEAD
is applied to the rows within each partition to retrieve the value from the specified offset.
MySQL LAG Function
MySQL LAG is an analytical function that allows you to access the value of a column from the previous row in a dataset without having to write complex SQL queries. The basic syntax for the LEAD function in MySQL looks like this:
LAG(expression, offset, default) OVER ( [PARTITION BY partition_expression, ... ] [ORDER BY order_expression [ASC|DESC], ... ] )
expression
- is a parameter that specifies the column or expression to retrieve the value for.offset
- specifies the number of rows to look ahead.default
- is an optional value that is returned if there are no rows available for the specified offset.PARTITION BY
andORDER BY
clauses are used to define the partitions and sort order for the rows, respectively.LAG
is applied to the rows within each partition to retrieve the value from the specified offset.
MySQL FIRST_VALUE and LAST_VALUE Window Functions
The FIRST_VALUE and LAST_VALUE MySQL analytical functions can come in handy for cases when you need to retrieve the first and last value of an ordered set of data within a specified window. These functions are often used in combination with the OVER clause, which partitions the result set into groups and applies the function to each group separately.
FIRST_VALUE
As the name suggests, this function returns the first value in the specified window. The basic syntax looks like this:
FIRST_VALUE(expression) OVER ( [PARTITION BY partition_expression, ... ] ORDER BY sort_expression [ASC|DESC], ... [ROWS {UNBOUNDED|n} {PRECEDING|FOLLOWING}] )
expression
- is a parameter that specifies the column or expression to retrieve the value for.PARTITION BY
(optional) - is used to divide the result set into partitions or groups.ORDER BY
- specifies the column or expression to order the result set by.ROWS
- specifies the range of rows to include in the window.
LAST_VALUE
This function, on the other hand, returns the last value in the specified window. The LAST_VALUE syntax is similar to the FIRST_VALUE syntax, but with the addition of the ROWS BETWEEN clause:
LAST_VALUE(expression) OVER ( [PARTITION BY partition_expression, ... ] ORDER BY sort_expression [ASC|DESC], ... ROWS BETWEEN {UNBOUNDED|n} {PRECEDING|FOLLOWING} AND {UNBOUNDED|n} {PRECEDING|FOLLOWING} )
expression
- is a parameter that specifies the column or expression to retrieve the value for.PARTITION BY
(optional) - is used to divide the result set into partitions or groups.ORDER BY
- specifies the column or expression to order the result set by.ROWS BETWEEN
- specifies the range of rows to include in the window, with the first argument specifying the lower bound and the second one - the upper bound.UNBOUNDED
- is a keyword that means that there is no lower or upper bound.n
- is a keyword that specifies the number of rows before or after the current row.
Best Practices for Using LEAD and LAG Analytical Functions
As we have discussed earlier in this article, LEAD and LAG functions are powerful tools for analyzing data in MySQL as they allow you to access data from previous or next rows in a result set, making it easier to perform calculations and comparisons. We have looked into the theory of the matter, and now it is time to apply this knowledge in practice. We will be using a convenient MySQL GUI editor - dbForge Studio for this purpose. Below, you will find examples of the best practices for using analytical functions:
Example 1: The LEAD function
Suppose you have a table named payment that contains information about the payments made by your customers, including the date of the sale and the amount paid. Let us also assume you need to calculate the difference in the amounts paid between each day and the next one. You can use the LEAD function to accomplish this. The query will look like this:
SELECT payment_date, amount, LEAD(amount) OVER ( ORDER BY payment_date) - amount AS difference FROM payment p ORDER BY payment_date;
In this query, we use the SELECT command to extract the payment_date and amount columns from the payment table. Then, we use the LEAD function in order to get the amount of the payments made on the next day. By subtracting the current day's amount from the one from the next day, we get the difference displayed in the difference column. LEAD is used along with the OVER() clause to ensure that the function returns the amount paid on the next day.
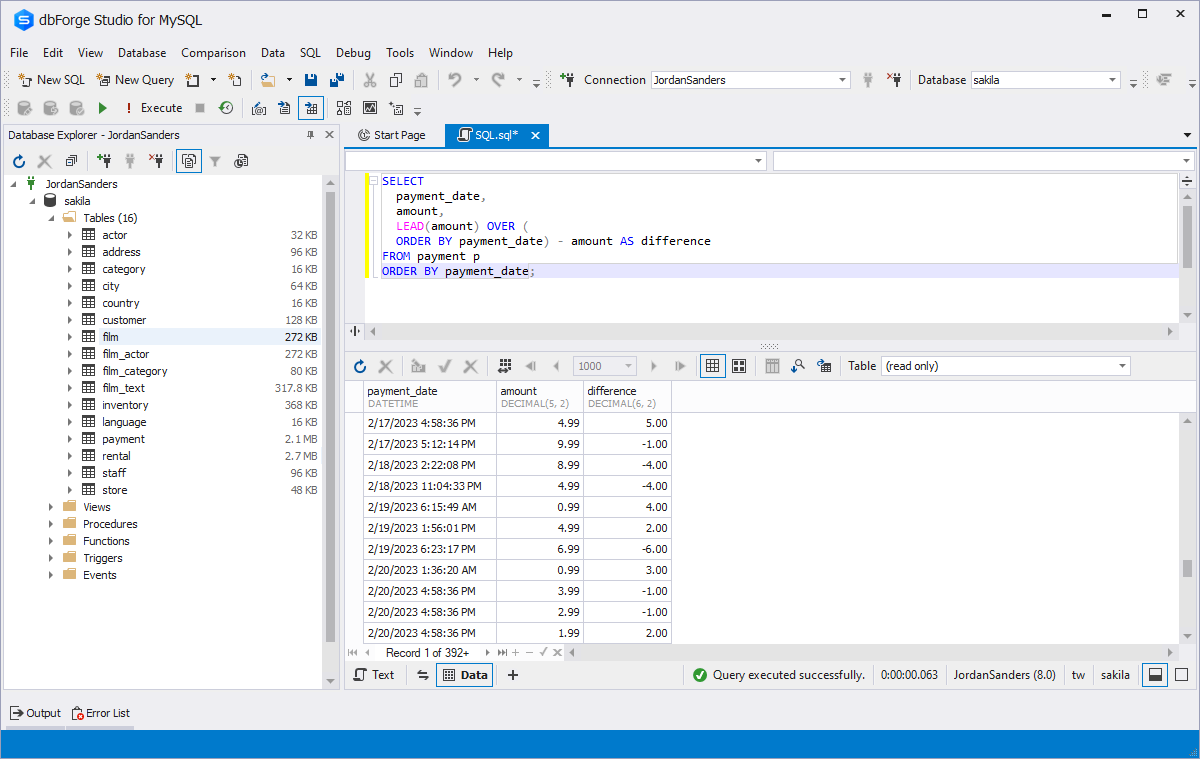
Example 2: The LAG function
Now, let us use the LAG function in the same field conditions:
SELECT payment_date, amount, LAG(amount) OVER ( ORDER BY payment_date) - amount AS difference FROM payment p ORDER BY payment_date;
Similar to the previous example, we can use the SELECT command to extract the payment_date and amount from the payment table. However, in this case, we use the LAG function to retrieve the amount of payments made on the day before. By subtracting the previous day's amount from the current day's amount, we can calculate the difference, which is then displayed in the difference column.
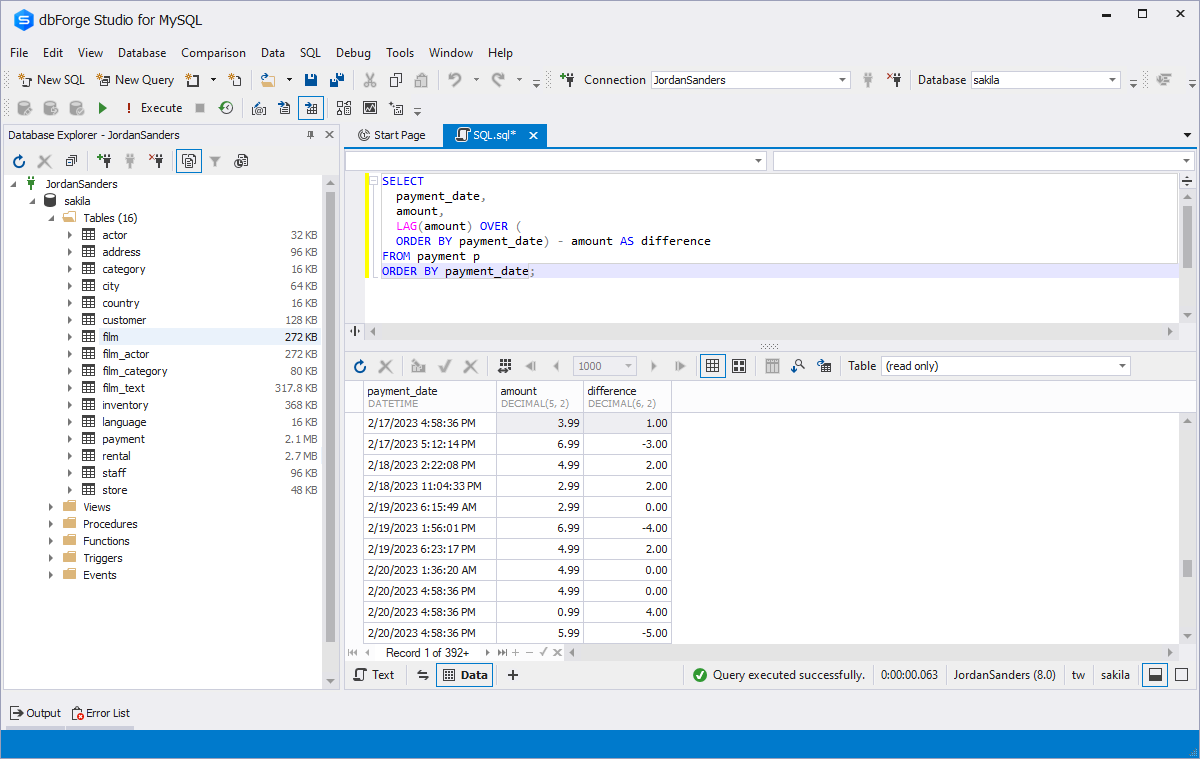
Example 3: The FIRST_VALUE function
In this example, we will describe how to use the FIRST_VALUE function in MySQL. Let's consider a film rental business where the staff uses a table called film to keep track of rentals. This table stores all the information regarding films available for rent and includes the film ID, title, description, release year, rental duration, rate, replacement cost, and more. If we want to retrieve the title and rental_rate of the cheapest film in the table, the query will look like this:
SELECT title, rental_rate, FIRST_VALUE(title) OVER ( ORDER BY rental_rate) AS cheapest_rental FROM film;
The query will return a result set that includes the title and rental rate for each film, as well as the title of the cheapest film in the cheapest_rental column. The first row of the result set will contain the title of the film with the lowest rental rate in the entire table.
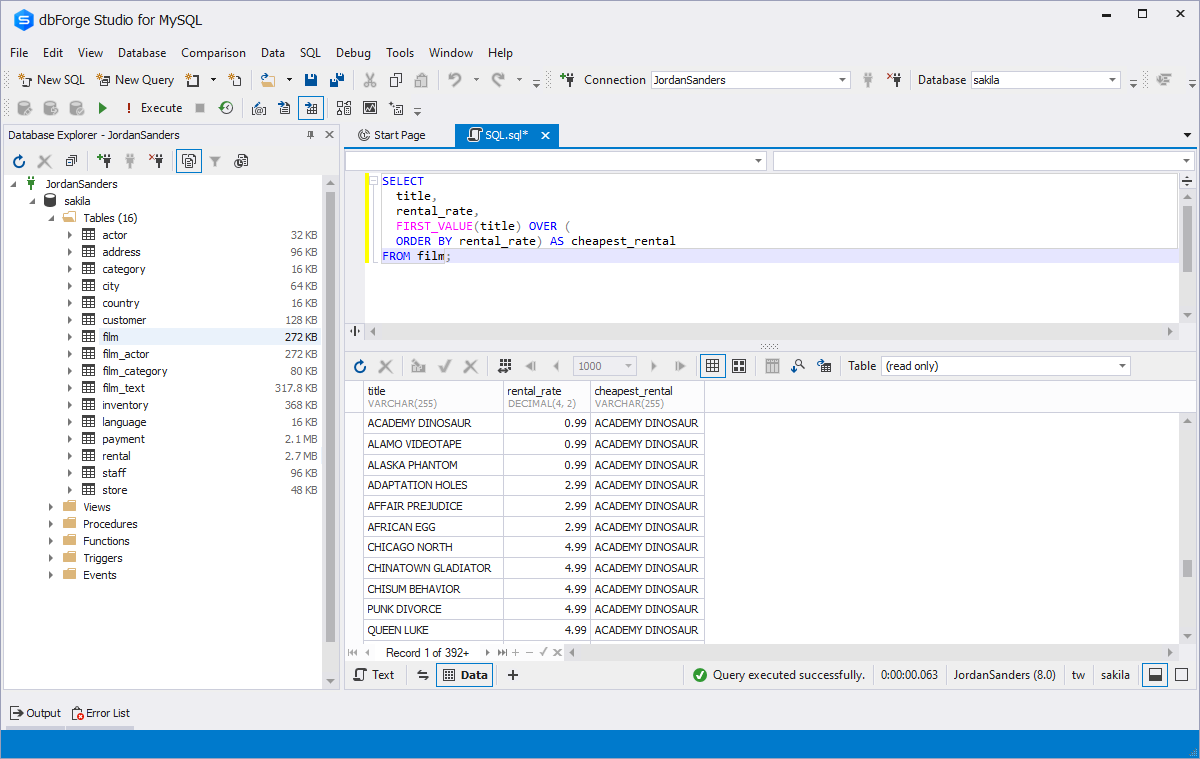
Example 4: The LAST_VALUE function
The last example depicts a situation when we need to retrieve the most expensive film available for rental:
SELECT title, rental_rate, LAST_VALUE(title) OVER ( ORDER BY rental_rate) AS highest_rental FROM film WHERE rental_rate <= 4.99;
The query will return a result set that includes the title and rental rate for each film, as well as the title of the film with the highest rental rate in the highest_rental column. The last row of the result set will contain the title of the film with the highest rental rate in the entire table. The WHERE clause is used to filter the result set based on a condition. Specifically, it filters the results to include only the films with a rental rate less than or equal to 4.99.
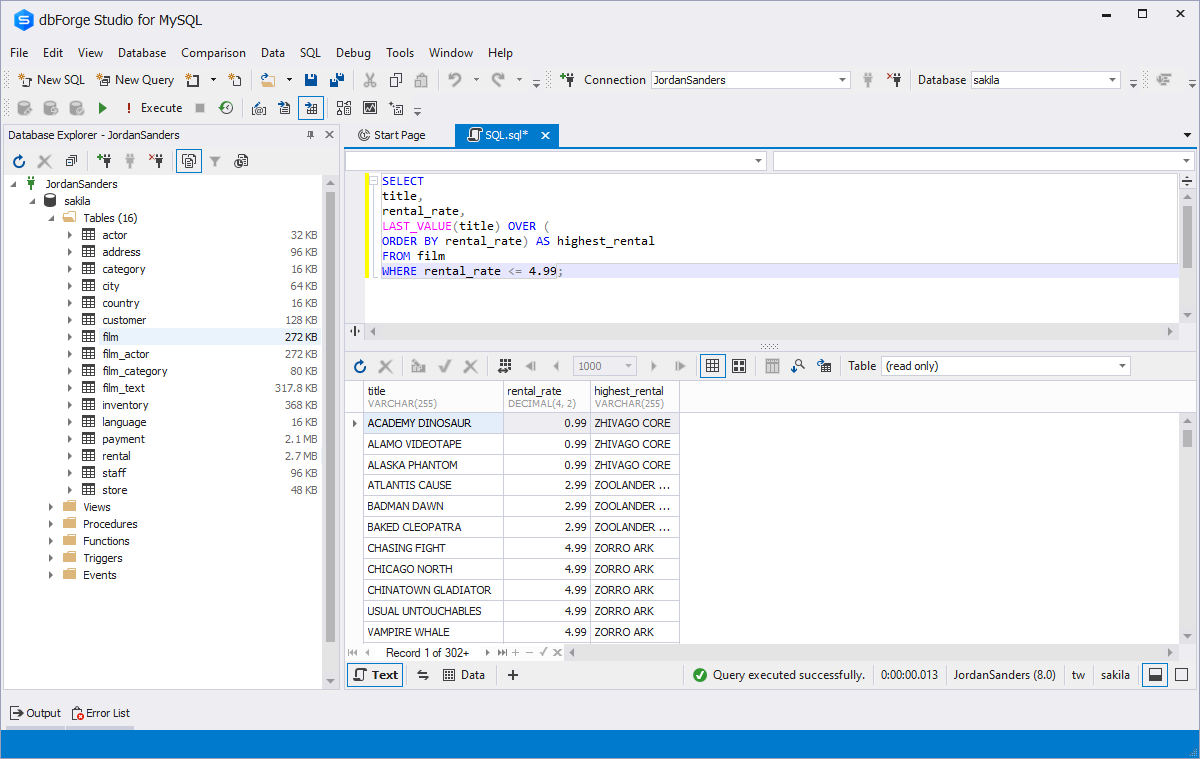
Conclusion
You now have the skills to apply various analytical functions like LEAD, LAG, FIRST_VALUE, and LAST_VALUE in MySQL to make your database development and management easier. Give the best IDE on the market a try: download dbForge Studio for MySQL for a free 30-day trial and experience how effective it can be in streamlining your daily activities.
We use cookies to provide you with a better experience on the Devart website. You can read more about our use of cookies in our Cookies Policy.
Click OK to continue browsing the Devart site. Be aware you can disable cookies at any time.